Webdriver を使用して Web アプリケーションを自動化しようとする場合、通常はページ上の HTML 要素を見つけることから始めます。 そして、それらを見つけるための正しい戦略を選択することになります。
以前のブログ記事で、Webdriver の findElement() コマンドの例をたくさん紹介しました。
次に、これらのメソッドと一緒に使用できるさまざまなロケーター戦略について説明します。
さらに、findElement と findElements の違いを明確に理解する必要があります。
今日取り上げるトピックのリストは以下のとおりです。
Table of Content.
- Firefox のインスペクタと FirePath ツールを使用してロケータを見つけます。
- findElement と findElements メソッドの違い
- ロケータにアクセスするための複数の By 戦略を理解する
- By.id().
- By.name().
- By.className().
- By.tagName().
- By.link() と By.partialLinkText().
- By.cssSelector().
- By.xpath().
では、まずアニメーションGIFでFirefoxのインスペクタとFirePathツールのデモを見てから、findElementメソッドとfindElementsメソッドの主な違いを確認していきましょう。
1- Firefox のインスペクタと FirePath ツールを使用したロケータの検索
Firefox の内部インスペクタ ツールを使用して固有のロケータを検索する方法を紹介するために、小さなアクション セットをキャプチャしました。
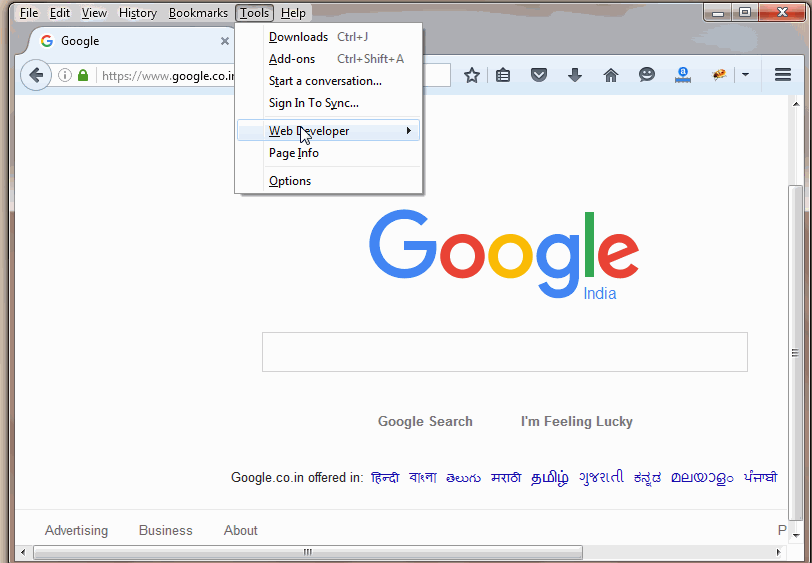
2- findElementとfindElementsメソッドの違い
findElement()メソッド
- このコマンドを使って、Webページ上の任意の単一の要素にアクセスすることができます。
- 指定されたロケータの最初にマッチする要素のオブジェクトを返します。
- 要素の発見に失敗した場合は、NoSuchElementException例外を投げます。
- 構文は次のとおりです。
- WebElement user = driver.findElement(By.id(“User”));
findElements()メソッド
- まず、使い方が非常に限られているため、頻繁には使用しません。
- 指定されたロケータに一致するすべての要素のリストを返します。
- 要素が存在しないか、ページ上で利用できない場合、戻り値は空のリストになります。
- 構文は次のとおりです。
- List<WebElement> linklist = driver.findElements(By.xpath(“//table/tr”));
TOC
コード例 – findElementおよびfindElementsメソッドです。
ここでは、findElementとfindElementsメソッドのわかりやすく実用的な例を示します。
import org.openqa.selenium.By;import org.openqa.selenium.WebDriver;import org.openqa.selenium.WebElement;import org.openqa.selenium.firefox.FirefoxDriver;public class findElementTest {public static void main(String args) throws Exception {// Start browserWebDriver driver = new FirefoxDriver();// Maximize the windowdriver.manage().window().maximize();// Navigate to the URLdriver.get("//www.techbeamers.com");// Sleep for 5 secondsThread.sleep(5000);// Here, see how the XPath will select unique Element.WebElement link = driver.findElement(By.xpath("'//table/tr/td/a"));// click on the linklink.click();}}
import java.util.Iterator;import java.util.List;import org.openqa.selenium.By;import org.openqa.selenium.WebDriver;import org.openqa.selenium.WebElement;import org.openqa.selenium.firefox.FirefoxDriver;public class findElementsTest {public static void main(String args) throws Exception {// Launch browserWebDriver driver = new FirefoxDriver();// Maximize windowdriver.manage().window().maximize();// Navigate to the URLdriver.get("//www.techbeamers.com");// Sleep for 5 secondsThread.sleep(5000);// Here, the code below will select all rows matching the given XPath.List<WebElement> rows = driver.findElements(By.xpath("//table/tr"));// print the total number of elementsSystem.out.println("Total selected rows are " + rows.size());// Now using Iterator we will iterate all elementsIterator<WebElement> iter = rows.iterator();// this will check whether list has some element or notwhile (iter.hasNext()) {// Iterate one by oneWebElement item = iter.next();// get the textString label = item.getText();// print the textSystem.out.println("Row label is " + label);}}}
TOC
3- ロケータにアクセスするための複数のBy戦略を理解する。
Webdriverは、findElement(By.<locator()>)メソッドを使用してWeb要素を参照します。 findElementメソッドは、<“By”>と呼ばれるロケータ/クエリーオブジェクトを使用します。 By “戦略には様々な種類があり、必要に応じて利用することができます。
3.1- By ID.
Command: driver.findElement(By.id(< element ID>))
このストラテジーによると、Byメソッドはid属性の値にマッチする最初の要素を返します。 一致する要素が見つからない場合は、NoSuchElementExceptionが発生します。 通常、IDはユニークな値を持っているので、要素のIDを使用することは、要素を見つける最も望ましい方法です。 しかし、開発段階にある大規模なプロジェクトでは、開発者は一意でないIDや自動生成されたIDを使用することになるかもしれません。
例:
<input>
// Java example code to find the input element by id.WebElement user = driver.findElement(By.id("TechBeamers"));
TOC
3.2- By Name.
Command: driver.findElement(By.Name(<element-name>))
By.Name()は、要素を見つけるもうひとつの便利な方法ですが、Idで見たのと同じ問題が発生しがちです。
この戦略では、<By.Name()> メソッドが name 属性に一致する最初の要素を返すようにします。
Example:
<input name="TechBeamers">
// Java example code to find the input element by name.WebElement user = driver.findElement(By.name("TechBeamers"));
3.3- By Class Name.
Command: driver.findElement(By.className(<element-class>))
このメソッドは、”class “属性で指定された値にマッチする要素を与えます。
Example:
<input class="TechBeamers">
// Java example code to find the input element by className.WebElement user = driver.findElement(By.className("TechBeamers"));
TOC
3.4- タグ名で。
Command: driver.findElement(By.tagName(<htmlタグ名>))
このメソッドを使って、指定したタグ名にマッチする要素を探すことができます。 このメソッドは、タグ内のコンテンツを抽出したいときや、タグ要素に対して何らかのアクションを実行したいときに呼び出すことができます。
例:
<form action="viewuser.asp" method="get"> Name: <input type="text" name="name"><br> Age: <input type="text" name="age"><br></form><button type="submit" form="userform" value="Submit">Submit</button>
WebElement form = driver.findElement(By.tagName("button"));// You can now perform any action on the form button.form.submit();
TOC
3.5- By LinkText or PartialLinkText.
Command: driver.findElement(By.linkText(<link text>))
driver.findElement(By.partialLinkText(<link text>))
この方法を使うと、リンクや部分リンクの名前を持つ「a」タグ(Link)の要素を追跡することができます。
Example:
<a href="#test1">TechBeamers-1</a><a href="#test2">TechBeamers-2</a>
// Java example code to find element matching the link or partial link text.WebElement link = driver.findElement(By.linkText("TechBeamers-1"));assertEquals("#test1", link.getAttribute("href"));// OrWebElement link = driver.findElement(By.partialLinkText("TechBeamers-2"));assertEquals("#test2", link.getAttribute("href"));
TOC
3.6- cssSelectorで。
Command: driver.findElement(By.cssSelector(<css-selector>))
この方法では、CSSセレクタを使って要素を探すことができます。
例:
<input class="email" type="text" placeholder="[email protected]"><input class="btn btn-small" type="submit" value="Subscribe to our blog">
// Java code example for using cssSelector.WebElement emailText = driver.findElement(By.cssSelector("input#email"));//OrWebElement emailText = driver.findElement(By.cssSelector("input.email"));WebElement subscribe = driver.findElement(By.cssSelector("input"));
上記の例とは別に、Htmlの属性に対して部分一致を行うこともできます。
1) ^= as in input means Starting with the given string. 2) $= as in input means Ending with the given text. 3) *= as in Input means Containing the given value.
TOC
3.7- By XPath.
Command: driver.findElement(By.xpath(<xpath>))
XPathクエリを使って要素を探したいときにこの方法を試してみてください。 XPath はドキュメント オブジェクト モデルを横断する方法で、XML ドキュメントの特定の要素、属性、またはセクションを選択する機能を提供します。
Example:
// Java code example for XPath.// Absolute path.WebElement item = driver.findElement(By.xpath("html/head/body/table/tr/td"));// Relative path.WebElement item = driver.findElement(By.xpath("//input"));// Finding elements using indexes.WebElement item = driver.findElement(By.xpath("//input"));// Finding elements using attributes values.WebElement item = driver.findElement(By.xpath("img"));// XPath starts-with() example.// => input// XPath ends-with() example.// => input// XPath contains() example.// => input
TOC
Footnote.
私たちがこの記事を書いたのは、findElement および findElements メソッドの詳細を知ってもらいたかったからです。 もう 1 つのコンセプトは、異なる By.locator() 戦略を選択することでした。 これらの概念は、リアルタイムのWebアプリケーションで練習することによってのみ習得することができます。
この記事では、Webdriver コマンドを練習するための多くのデモ Web サイトをご覧いただけます。
この知識を応用して、より優れた自動化ソリューションを作成していただけることを期待しています。